What's new about vue.3
Updated September 28, 2021 | 5 min read
Vue is a progressive javascript framework for UIs (user interfaces), the most starred in Github, and it is commonly used in frontend development next to React and Angular.
In september 2020, a new major release came out: VUE 3.0.
Let take a look at the new features:
1- Composition API
If you're familiar with Vue 2 you'll be quite used to what is called the options API which looks like this :
// Example of a search + sorting component
export {
data():{
return {
// Data for searching
// Data for sorting
}
},
methods: {
// function for search
// function for sorting
}
}
Now all of your data, your computed properties, your watches, your methods and so on are containing their own options within the Vue object.
And while this is feels nice and intuitive, VUE 2 have 3 main problems:
- First is as your components grow and get bigger, they become less readable and therefore less maintainable.
- Second is that as you reuse pieces of code across components, each of these code reuse patterns in Vue 2 has its drawbacks.
- Third is that you Vue 2 has limited typescript support, there's ways to write it with typescript but they're not completely optimal.
So instead of organizing our features by component options (and there is quite a few in Vue), we can do something like this:
// Example of a search + sorting component
export {
setup():{
// Setup method
return {...useSearch(),...useSorting()}
}
},
function useSearch(){
// composition function for search
},
function useSorting(){
// composition function for sorting
}
}
Now our code is organized by logical concerns, therefore more readable and maintainable.
Vue 3 makes it possible by introducing the setup function and the composition functions (which we will dive into their syntax later in another article).
2- Faster and smaller
Vue team have rewritten the entire framework to be faster and smaller than Vue 2. So let's go over some specific ways that the team have been able to implement it.
They utilized a method called tree shaking and it basically removes dead code when compiling, so that when you compile your project for production, you will be leaved with the slimmest version of your app possible. Smaller project size means faster page load for your users.
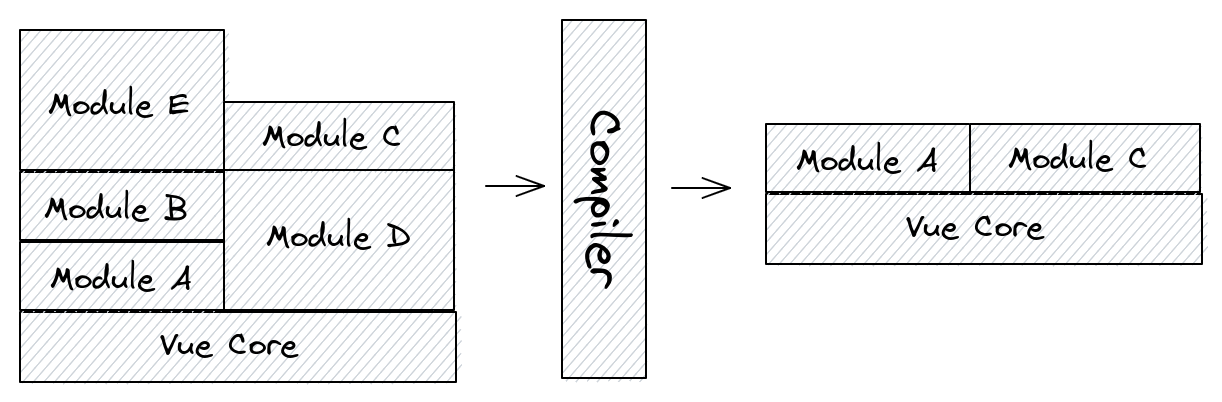
Also vue has made its rendering faster is by implementing what it's called: a compiler informed virtual DOM.
Now Vue is 41% lighter, has 55% faster renders and 133% faster updates and consumes up to 54% less memory. For full statistics visit this link.
3- Teleport
In simple terms, teleport allows us to render components wherever we want within a page. Most of the time a component should be rendered naturally wherever its place is in the template, but specific features make more sense to be rendered outside of where it logically sitting in our code.
To show an example of this, let's look at a common feature of web applications today the modal.
<!-- App.vue -->
<template>
<body>
<div class="relative">
<h3> Vue 3 Modal </h3>
<div>
<modal-button>
</div>
</div>
</body>
</template>
In this example the modal button component would allow us to click a button and open a modal on the page, but the problem with this is that the modal generated in the component is now nested deeply within the application, while it would make most sense to sit right at the top as a direct child of the body.
<!-- modalButton.vue -->
<template>
<button @click="modalOpen = true">Open modal</button>
<div v-if="modalOpen">
<div>
I am a modal !
<button @click="modalOpen = false">Close</button>
</div>
</div>
</template>
Teleport allows us to solve this problem easily by wrapping a modal in a teleport tag and then telling it where to render. It's as simple as that, now our modal will be rendered on the page as a child of the body, allowing it to be placed above the rest of the application on the page.
<!-- modalButton.vue -->
<template>
<button @click="modalOpen = true">Open modal</button>
<teleport to="body">
<div v-if="modalOpen">
<div>
I am a modal !
<button @click="modalOpen = false">Close</button>
</div>
</div>
</teleport>
</template>
4- Script setup (experimental)
Some new features were announced, but they are still experimental like the script setup property.
Let take a simple example:
<script>
import { ref } from 'vue';
export default {
setup() {
const count = ref(0);
const inc = () => count.value++;
return {
count,
inc,
};
},
};
</script>
This code is already clean and straight forward, but with this new property we can simplify it a bit further like this:
<script setup>
import { ref } from 'vue';
export const count = ref(0);
export const inc = () => count.value++;
</script>
5- Style vars (experimental)
Using the vars attribute, allows us to reference css variables that are being generated from our component state.
<template>
<div class="text">hello</div>
</template>
<script>
export default {
data() {
return {
color: 'red',
};
},
};
</script>
<style vars="{ color }">
.text {
color: var(--color);
}
</style>
Take a look at this example, this feature now allows us to dynamically update our css stylings using our state logic by referencing the state variable in the vars attribute and then placing that inside a normal css variable reference.
6- Final notes
And that's it, that's all the major features that have arrived with V3. Of course there are even more changes that we didn't go over in this article, so if you have any more interest in every single tiny little detail that has been changed in Vue 3 go check out the documentation or the v3 release notes for a little bit more information.