The ultimate git guide
#web dev #coding tips
Updated January 9, 2021 | 6 min read
Git is the most commonly used version control system.
GitHub is a cloud-based hosting service that lets you manage Git repositories. It is widely used and it is the one I use myself.
Git allows developers to:
- Tracks the changes you make to files, so you have a record of what has been done.
- Revert to specific versions should you ever need to.
- Makes collaboration easier, allowing changes by multiple people to all be merged into one source.
0- Terminology
- Snapshot: just like in photography, a snapshot in computer science represents a moment (timestamped), where the system was in a certain state.
- Commit: In short, a commit is a snapshot of your Git repository at one point in time.
- Push request: synchronizing changes from a local repository (folder) to a remote one.
- Remote vs Local: Remote refers to the version of the project sitting on the cloud (Github in this case). Local refers to the version of the project that you have on your machine.
- The working tree: The working tree, or working directory, consists of files that you are currently working on, if there is nothing to commit then the git status will return a clean working tree.
1- New project
- After creating a new account on Github, and installing git locally from your Linux terminal:
apt-get install git
( for windows & mac check this link ), you need to configure the email and username:
git config --global user.name name #replace with your github name
git config --global user.email email #replace with your github email
- You can also edit the config file manually, as it will be set on your home repo (Linux based systems): ~/.gitconfig
- To start a new git project, create a new repository from your Github account without initializing it.
- Then in your local machine, cd to the project folder and do the following:
# init an empty repo with the branch main
git init -b main
# it tracks all the changes made to the folder
git add .
git commit -m "first commit"
# replace that with your actual repo link
git remote add origin https://github.com/user/repo.git
# you will be prompted to enter your github password
git push origin main
- Update As of 2022 password connections are no longer supported, you need to go to your account developers' settings and generate a token (with reading/write permissions).
- Most of the time there are files that you don't want to commit to production, for that create a .gitignore file in your root project folder, and fill it with the paths of files & folders that you want to ignore. You can also set up a global gitignore file to always include it in your projects, configure it once and use it:
git config --global core.excludesfile ~/.gitignore_global
- This site give you recommendations on what to ignore based on your system & IDE...
2- Git branches
- One of the powerful features of git is branches: you can think of them as a sequence of commits and the branch have a movable pointer, the sequence grows and the pointer moves forward with each commit.
- Every commit (circle) represents a snapshot of your project, at the time of the commit.
- Branches allow you to develop features, fix bugs, or safely experiment with new ideas in a contained area of your repository.
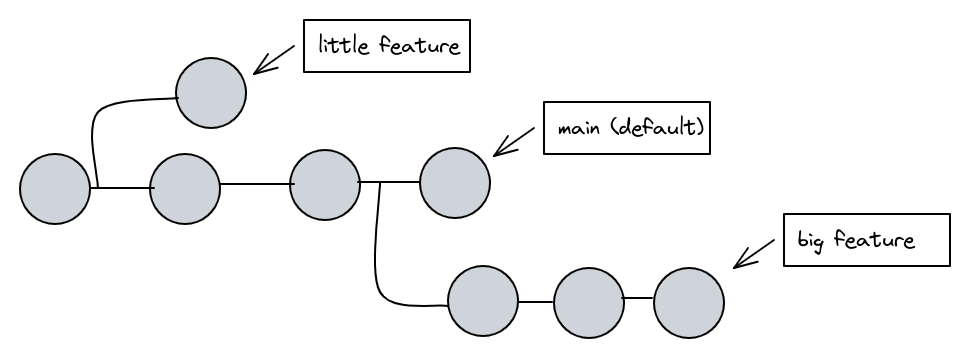
- The diagram above visualizes a repository with two isolated lines of development, one for a little feature, and one for a longer-running feature. By developing them in branches, it’s not only possible to work on both of them in parallel, but it also keeps the main branch free from questionable code.
- Ps: GitHub (and the industry at large) is moving to rename the default branch as main instead of master.
- Here are useful commands to work with branches:
# list all the branches in a project
git branch
# create a new branch with name test without checking out
git branch test
# delete the test branch if it has merged changes
git branch -d test
# Force delete even if test has unmerged changes
git branch -D test
# rename the current branch to test
git branch -m test
# list all the remote branches
git branch -a
# checkout to the branch test
git checkout test
- When you create a new branch, git only creates another pointer to the last commit, you need to checkout to the branch and add new code and commit.
3- Revert to previous
- So you committed changes, just to realize that you screwed up, how to fix it now?
- You can either reset:
- Affect only the remote repo, not the local one:
git reset --soft HEAD~1
- The most direct, dangerous, and frequently used option. When passed --hard The Commit History ref pointers are updated to the specified commit:
git reset --hard HEAD~1
- This is the default operating mode. The ref pointers are updated. The Staging Index is reset to the state of the specified commit:
git reset --mixed HEAD~1
- Affect only the remote repo, not the local one:
- Or you can revert:
git revert HEAD~3
(Revert the changes specified by the fourth last commit in HEAD and create a new commit with the reverted changes).
💡 Remove not commited changes from a branch and add them to a new one:
git stash
git checkout -b new-branch
git stash popl
4- Contribute
- You want to create a local copy of an opensource project from Github:
# replace with the actual repo link
git clone https://github.com/user/repo.git
- You want to contribute to a project: - Find a project worth working on. - Fork it and clone it with
git clone
. - Open the project in your favourite IDE & create a new branch withgit checkout -b NewBranch
. - Make the changes to the project, thengit add
&git commit -m "message"
&git push origin NewBranch
. - Now go the remote repository and open a pull request , github will automatically detect if merging your new branch to the main one will cause any conflicts. - Wait for the admin code review, if approved cool 🎉 congratulations, if not refactor you code & repeat :)
5. Final notes
- You want to visualize your commits history:
git log
- Git has become an industry standard today, and while this article is not an in-depth tutorial about the system, I hope it gets you starting, and don't forget to check the external links and do your own research and experiment!
- This was a very quick introduction about branches, to learn about them in detail please visit: https://learngitbranching.js.org/